Types of Addressing Modes
Various types of addressing modes are used to specify an operand in an instruction. The choice of addressing mode can affect the efficiency and flexibility of a computer’s architecture.
Note: Before learning about the types of addressing modes, it is important to know computer instructions.
Types of Addressing Modes
In computer architecture, there are the following types of addressing modes.
- Implied / Implicit Addressing Mode
- Stack Addressing Mode
- Immediate Addressing Mode
- Direct Addressing Mode
- Indirect Addressing Mode
- Register Direct Addressing Mode
- Register Indirect Addressing Mode
- Relative Addressing Mode
- Indexed Addressing Mode
- Base Register Addressing Mode
- Auto-Increment Addressing Mode
- Auto-Decrement Addressing Mode
In this article, we will discuss these addressing modes in detail.
1. Implied Addressing Mode
Implied Addressing Mode is called ‘Implicit’ or ‘Inherent’ addressing mode.
- For implied addressing modes, the operand (data) is directly given and we do not need to find it.
- It is mainly used for Zero-address (STACK-organized) instructions.

Examples of zero address instructions are
- ADD (it takes the previous two values from the stack and adds them).
- CLC (used to reset the Carry flag to 0)
2. Stack Addressing Mode
The operand is found from the top of the stack in stack addressing mode.
Example of Stack Addressing Mode
ADD Operation in Stack organization is an example of stack addressing mode.
- Take the top two values from the top of the stack
- Apply ADD instruction
- The obtained result is again placed at the top of the stack
3. Immediate Addressing Mode
It contains a constant value as an operand which is the identification of immediate addressing mode.
- We do not need to store the value of the constant in memory or register.
- The size of the constant must be equal to or less than the size of the operand field in the instruction.
- It is a faster mode than others because the operand is specified in the instruction. We can directly use it from the instructions. So, No extra computations are required to calculate effective address (EA).
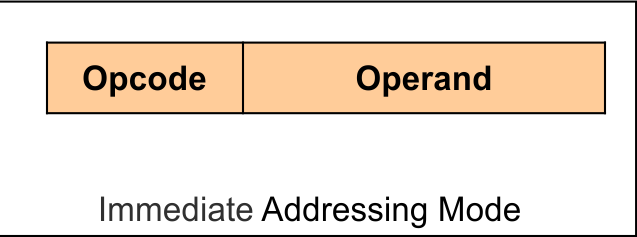
Examples of Immediate Addressing Mode
- ADD 10 (AC = AC+10)
- MOV AL, 30H (move the data (30H) into the AL register)
4. Direct Addressing Mode
Direct addressing mode is also called an absolute addressing mode. It involves the following
- The operand of instruction contains the address of the memory location.
- All computer hardware or instruction sets support direct and indirect addressing modes.
Example of Direct Addressing Mode
ADD AL,[0302] //add the contents of address 0302 to AL
Problem with Direct Addressing Mode
In the case when we have a fixed 16 bits ( where, 4 bits for opcode and 12 for operand) instruction size, Then we cannot give the operand address greater than 12 bits.
To resolve this issue, we use indirect register addressing mode. We give the address of the register in 12 bits, and in that register, we provide the address of the memory location greater than 12 bits.
5. Indirect Addressing Mode
In indirect mode, the address field in the instruction contains the location of the register or memory where the effective address (EA) of the operand is present. EA leads to actual operand (data).
- Two references of memory are required to fetch the required data. The first address leads toward the second address and the second leads toward actual data.
- Pointers or variables are used to contain the address of another variable.
Disadvantage: Computations are increased.
Effective address (EA): The address where the actual operand is found is called an effective address.
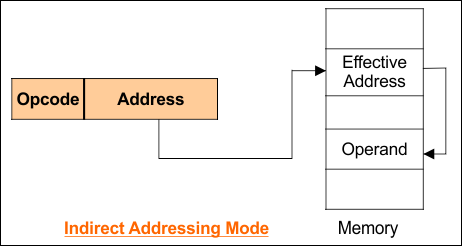
Example of Indirect Addressing Mode
ADD X in indirect addressing mode will perform in the following way
AC ← AC + [[X]]
6. Register Direct Addressing Mode
The address field of the instruction specifies a CPU register that contains the operand.
- There is no need for memory access to fetch the operand.
Example of Direct Addressing Mode
ADD R in register direct addressing mode will perform in the following way
AC ← AC + [R]
7. Register Indirect Addressing Mode
The address field of the instruction specifies a CPU register, which provides the effective address of the operand.
- There is only one memory reference is required to fetch the operand.
Example of Indirect Addressing Mode
ADD R in register indirect addressing mode will perform in the following way
AC ← AC + [[R]]
Advantage of Indirect Addressing Mode
Addressing modes reduced the instruction size. For example, If we have 4GB memory, then to fetch any operand from this memory, we require a 32-bit address. In this way, a 32-bit operand address will increase instruction size. To reduce instruction size, we use registers generally 64; these registers store the address of that operand in any register. In this way, only 6 bits are required for instruction to access the actual operand from memory. It’s all the explanation of register indirect addressing mode.
Disadvantage of Indirect Addressing Mode
Register indirect addressing mode requires three read operations to access an operand. So, more computation is needed.
8. Relative Addressing Mode
The effective address of the operand is obtained by adding the address of the program counter with the address part of the instruction.
Effective address = Value of PC + Address part of the instruction
Explanation of Relative Addressing Mode
In the instruction, We provide the value of offset instead of providing the actual address in the address field. The offset value is added to the PC value to get the actual address of the operand.
- For example, if the Offset value (displacement value) is 49 and the program counter value is 501 then we will find the operand in memory at 550 address.
Advantage of Relative Addressing Mode
The offset reduces the instruction size because we give offset value in instruction rather than the actual address. As we mentioned, offset (BR 49) rather than BR 550.
9. Indexed Addressing Mode
The operand field contains the starting base address of the array-memory block, and the general register (index-register) field will contain the index value.
- Adding a base address and index register will give the actual physical address of the operand.

So, EA= base address + index register
The address is fixed for the base register. However, the index register value changed as required.
10. Base Register Addressing Mode
The effective address of the operand can be found by adding the value of the base register with the address part of the instruction.
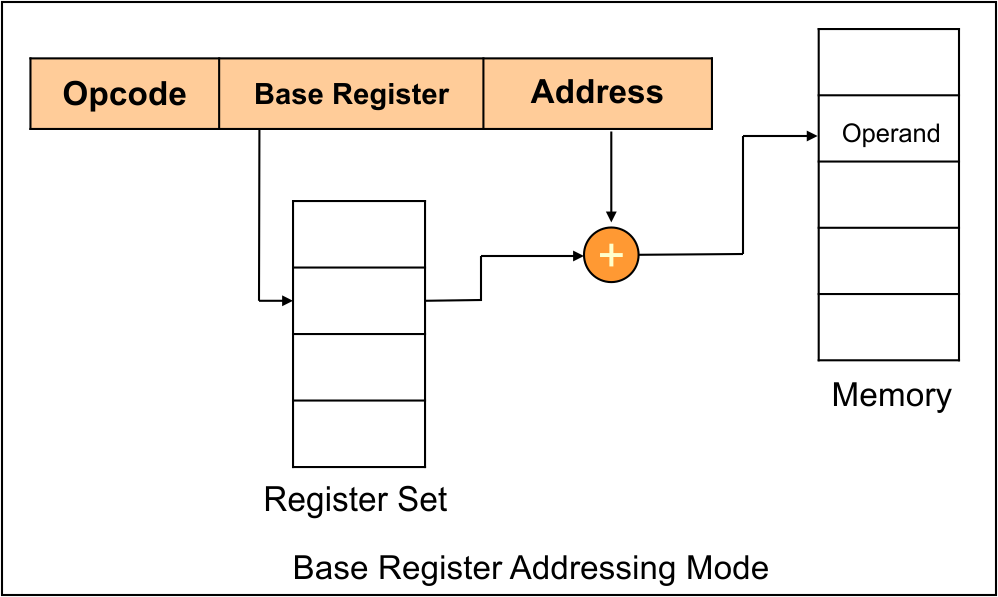
Effective address = Content of Base Register + Address part of the instruction
11. Auto-Increment Addressing Mode
This addressing mode is a special case of Register Indirect Addressing Mode. Auto-increment and decrement are used to access the data in sequence from memory.
Effective Address of the Operand = Content of Register
After accessing the operand, the value of the register is automatically incremented by step size ‘d’. The size of ‘d’ depends on the size of the operand. If the operand size is 4 bytes, then the size of “d” will be 4 bytes. To access the operand, Only one memory reference is required.
Explanation of Auto-Increment Addressing Mode
We only apply increments and fetch the next value from memory in each clock pulse. So, we can use a counter loop on memory.
Only starting addressing is placed in the register set as in R1, through computation we found the actual operand from memory. But the rest of the operands in memory are directly accessed through auto increment or decrement in register R1. For that, we do not need to do all computations like the first one. So, the fewer computations there are, the higher the speed.
Example of Auto-Increment Addressing Mode
- Add R1, (R2)+ // OR
- R1 = R1 +M[R2]R2 = R2 + d
12. Auto-Decrement Addressing Mode
This addressing mode is also a special case of Register Indirect Addressing Mode in which
Effective Address of the Operand = Content of Register – Step Size
The value of the register is decremented by step size ‘d’. The size of ‘d’ depends on the size of the operand. If the operand size is 4 bytes, then the size of d will be 4 bytes. To access the operand, Only one memory reference is required.
Example of Auto-decrement Addressing Mode
- Add R1,-(R2) //OR
- R2 = R2-dR1 = R1 + M[R2]
Addressing Modes Examples
Let’s discuss various examples of addressing modes
Addressing Mode Example: 01
An instruction is stored at location 300 with its address field at location 301. The address field has the value 400. A processor register R1 contains the number 200. Evaluate the effective address if the addressing mode of the instruction is.
- Direct
- Immediate
- Relative
- Register Indirect
- Index with R1 as the index register
Solution for given Example
1. Direct addressing mode
The address field contains the effective address in the direct address mode, the effective address is the address part of the instruction 400.
EA = 400
2. Immediate addressing mode
Operand value in the address field. In immediate mode, the second word of the instruction is taken as the operand rather than an address. The effective address in this case is 301.
EA = 301
3. Register Addressing Mode
The register contains the effective address of the operand. In register indirect mode, the effective address is 200, which is the content of R1.
EA = 200
4. Relative addressing mode
PC is added to the address part. In relative address mode, the effective address is 400+302 = 702. (PC = PC+2)
EA = 702
Note: The value in PC after the fetch phase and during the execute phase is 302.
5. Index addressing mode
The index register is added to the address part. The effective address are XR + 400= 200+400 = 600.
EA = 600
Table of all addressing modes with their effective address.
Addressing Mode Example: 02
let’s discuss several different types of questions for a better understanding of various types of addressing modes. Look at the following picture
The two-word instruction at address 200 and 201 is a “load to AC” instruction with an address field equal to 500.
- The first word of the instruction specifies the operation code and mode, and the second word specifies the address part.
- PC (Program counter) has the value 200 for fetching this instruction. The CPU register (R1) content is 400, and the content of an index register (XR) is 100.
- AC (Accumulator Register) receives the operand after the instruction is executed.
- The mode field of the instruction can specify any one of the number of modes.
Calculate the effective address for each possible addressing mode and the operand that must be loaded into AC.
Solution of given Question
Look at the following diagram which presents the solution of various addressing modes.
Following is the explanation of each addressing mode which tells us how we calculate the effective address and accumulator value.
1. Immediate addressing Mode
The operand value exists in the address field. The second word of the instruction is taken as the operand rather than an address, so 500 is loaded into AC. The effective address in this case is 201.
- Effective address (EA) = 201
- Accumulator Register (AC) = 500
2. Direct Addressing Mode
As the address field contains the effective address. The effective address is the address part of the instruction, 500 and the operand to be loaded into AC is 800. So,
- Effective address (EA) = 500
- Accumulator Register (AC) = 800
3. Indirect Addressing Mode
The Address field specifies the address where the effective address of the operand is stored in memory. The effective address is stored in memory at address 500. Therefore, the effective address is 800 and the operand is 300.
So,
- Effective address (EA) = 800
- Accumulator Register (AC) = 300
4. Register direct addressing Mode
As the register contains the operand and the operand is in R1, so 400 of R1 is loaded into AC. Therefore,
- Accumulator Register (AC) = 400
Note: There is no effective address in this case.
5. Register Addressing Mode
The register contains the effective address of the operand. The effective address is 400, equal to the content of R1, and the operand loaded into AC is 700.
- Effective address (EA) = 400
- Accumulator Register (AC) = 700
6. Auto – Increment Addressing Mode
It is totally similar to the register indirect addressing mode, except the register value is incremented after it is used. CPU register (R1) is incremented to 401 after the execution of an instruction.
- Effective address (EA) = 400
- Accumulator Register (AC) = 700
7. Auto–decrement addressing Mode
It is totally similar to the register indirect addressing mode, except the register value is incremented before it is used. CPU register (R1) is decremented to 399 before the execution of an instruction. The operand loaded into the AC is now 450.
- Effective address (EA) = 399
- Accumulator Register (AC) = 450
8. Relative Addressing Mode
PC is added to the address part. The effective address is 500 + 202 = 702 and the operand is 325.
- Effective address (EA) = 702
- Accumulator Register (AC) = 325
The value in PC after the fetch phase and during the execution phase is 202.
9. Indexed Addressing Mode
The index register is added to the address part. The effective address is XR + 500 = 100 + 500 =600 and the operand is 900.
- Effective address (EA) = 600
- Accumulator Register (AC) = 900
Applications of Addressing Modes
Following is a table of applications for various types of addressing modes.
Addressing Modes | Applications |
Immediate Addressing Mode | Initialize registers to a constant value |
Direct Addressing Mode and Register Direct Addressing Mode |
|
Indirect Addressing Mode and Register Indirect Addressing Mode | pointers implementations |
Relative Addressing Mode | To change the normal sequence of running instructions
For branch-type instructions |
Index Addressing Mode | Use in array implementation |
Base Register Addressing Mode | Implementation of relocatable code, i.e., relocation of program in memory even at run time |
Auto-increment Addressing Mode and Auto-decrement Addressing Mode |
For loop implementations, Traversing through arrays in a loop, Implementation of a stack, as push and pop |